GitHub Tutorial
by Xiurong Yu
Git vs. GitHub
Git: Keep “snapshots” of code & does not require github
Github: Stores code in the cloud, visually track changes, & requires git.
Initial Setup
- Make a github account.
- Go to www.github.com
- Click sign up in the upper right hand corner.
- Step 1 will ask you to fill in a username, email address, and password you want to use.
- Step 2 will ask you to choose a plan.
- Usually you would choose free plan unless you want to pay for the other one.
- Step 3 will ask you some question and you will answer them.
- Click submit once you completed all steps.
- Go to the email address you put down when signing up to github.
- You should see a message from github that askes you to verify your email address.
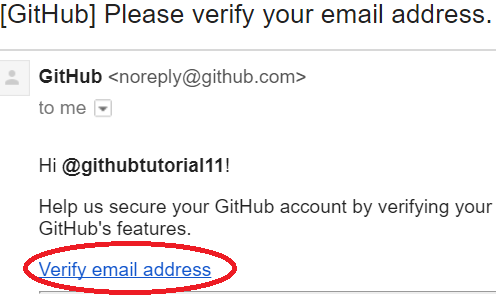
- Click on Verify email address and it will bring you to your github account. This means your email address is verified and you are able to access github features!
- SSH Setup
Setting up a SSH key allows you to create a connection with a remote server. In this case, you are trying to create a connection between github and cloud9.
Here are the steps to setting up SSH Key:
- In your github page, click on profile icon on the upper right hand corner and press settings.
- Then on the leftside bar (under personal setting), click on SSH and GPG Keys.
- Click New SSH key
- Go to your cloud9 account. Click on the gear icon on the upper right.
- Click on “SSH Keys” on the left.
- Copy the 2nd SSH Key to your github account.
- Your SSH is now set up!
Repository Setup
- Making a Repository (repo) and initialize git
Directory: Folder
Repository: When git is initialized, folders are called repo
Working Directory: where you’ll be doing all the work: creating, editing, deleting and organizing files
Staging Area: where you’ll list changes you make to the working directory
- Go to your github account that you have created. On the upper right hand corner, click the “+” sign and create a new respository.
- Give your repository a name (In this case I named it tutorial-repo). Once your repository is created, you should be seeing this
- Copy the SSH link on github; Make sure it’s the SSH link, not HTTP
- Go to your cloud9 account. On a new terminal/workspace and type in the following and paste the link after git clone.
git clone [link]
git clone: Creates a copy of a repo to a directory. In this case, we are cloning the remote repo (from github) to local workspace (in cloud9).
- Everytime you clone something, always cd into it
cd [name of the repo]
cd: Change directory (allows you to move into the directory you want to be in).
- Make a file in this repo and name it README.md (this will help you practice git add & git commit later on)
touch README.md
touch: makes a new file
- Now initialize git inorder to use git commands
git init
- You should be seeing something like this
username:~/workspace/reponame (master) $
git init: Creates a new, empty git repository. This is where git commands are allowed to be used
- Note: Do not ever git init in workspace because it is an empty git repository.
Error Handling
- If you already initialize it,
rm -rf .git
will help you remove it.
- rm -rf .git: Removes the .git repository completely (Undo git init)
- Notice the (master) is gone when you uninitialize git.

- First add and commit
-
Go to the readme file you creaded in cloud9 (the one you cloned from github)
cd [your repo]
- Open up the README.md file you created inside this folder and type in any message you want.
- Now go back to the command line, and add this file to staging area.
git add README.md"
- Git add: Adds files from the working directory to the staging area. Here are 3 ways you can use git add
git add [filename]
git add .
adds the current directory
git add --all
includes all changes, including deleted files
- Once you have added the file, commit it with a message that will help you reference what you did.
git commit -m "[message]"
Git commit -m: Takes a snapshot of the files on the stage.
- Message should be present tense and should describe the snapshot
- Now you have to set connection with your remote repo in github in order to push your commits. Use the following format
git remote add origin [URL]
git remote add origin [URL]: Sets connection with between the local repo and the remote repo, then, give it a short name
- Note: Once you added the remote origin, you no longer have to added next time unless you want the file to go to a diferent remote.
- you can find the URL under “…push an existing repository from the command line”
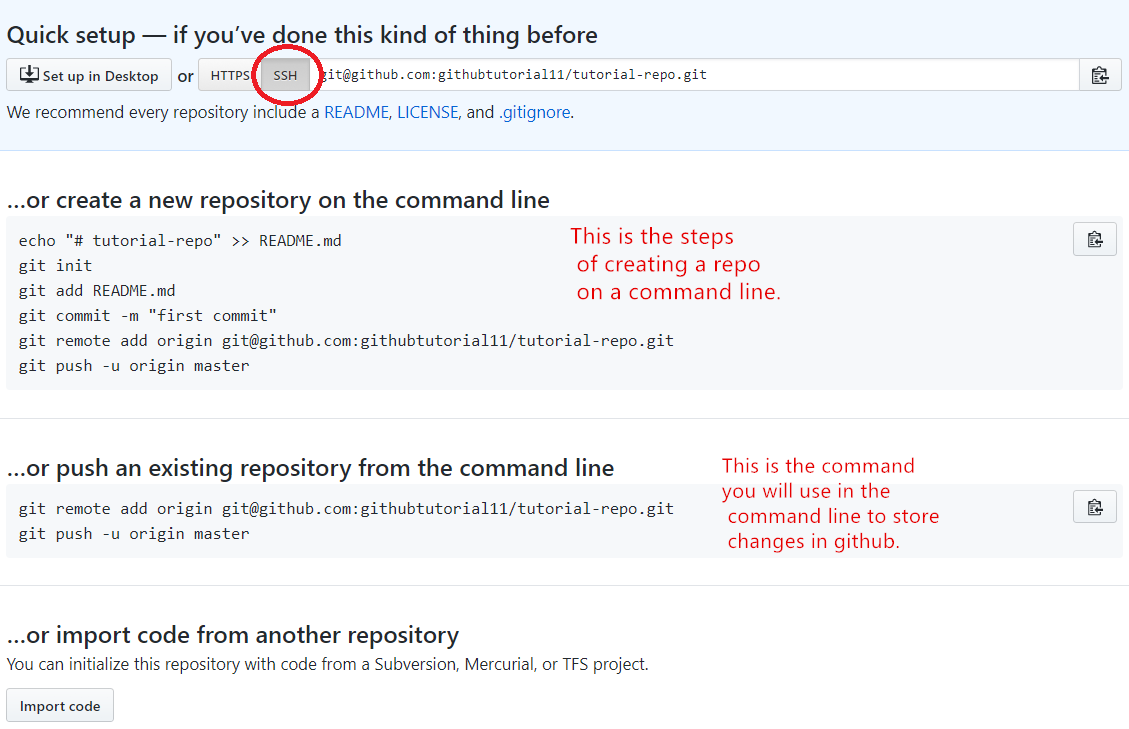
- Now type the second line of the code
git push -u origin master
git push -u origin master: allows you to push the changes you made in your local repo to the main branch (master) of the remote repository.
- This is only for the first time set-up; this means the next time you can just use
git push
to push into the same repo in github.
- You should be seeing the following after you push showing that you have successfully pushed your README file on github
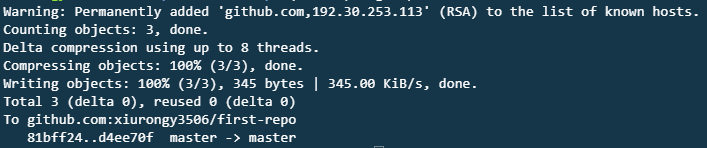
git push: Sends the commit from logo repo to remote repo
- Go back to your github account, open your repo, then press commits.
- You should be able to see the commit you just pushed! (For your repo, you should only see one commit)
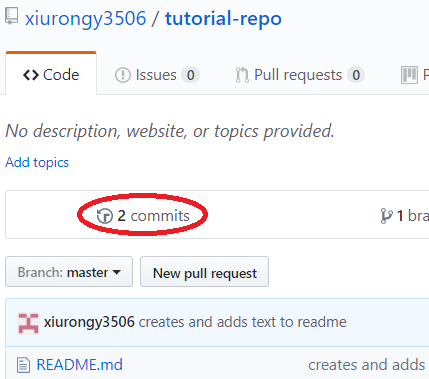
Workflow & Commands
If you want to commit another file to github(the remote repository), the process will be different. Let’s commit another message to the same README.md file we’ve been working on.
- Make sure you are on your repo in a command line (at cloud9)
- You should see
USERNAME: ~/workspace/[name of your repo] $
- Open up your README.md. You can use the following to help you open.
*
c9 README.md
- Add or delete anything in the text go back to your terminal.
- Now add your file to the staging area
git add README.md
- Use
git status
to check the state of your file
- You should see README.md in green showiing that it can be commited
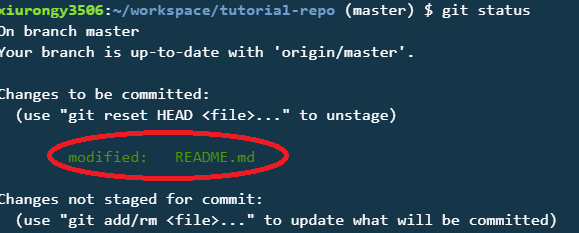
git status: Shows the state of file. Tells you the staged and unstagged file, and files that are not tracked.
- Now commit the file by stating what you did to the file `git commit -m “[text]”
- Push the commit to the remote. This time you don’t have to do
git remote add origin [URL]
or git push -u origin master
because it is a one time set-up that is already added. So just simply type git push
- You should still see something similar to
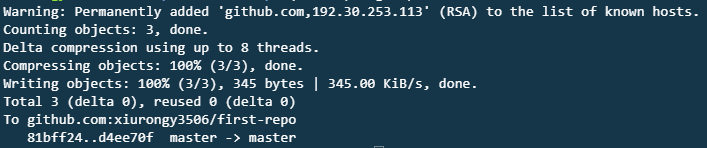
- Now you can go to github and check the commits in your repo. You should now see another commit being added to github!
Rolling Back Changes
undo edit/add/commit/push
- Undo edit
- Make sure you are in USERNAME:~/workspace/[name of repo]. If not cd into it.
- Go to your README.md and add some text.
-
Now use git status to check the stage of your file (try to use this command frequently to check your state of file)
git status
- Now notice the nessage above the README.md is red because you have not added to the staging area. Also, there are command listed for how to discard a change.
- Type in this command without using <> or ….
git checkout -- [file]
-
Now go open up your README.md
c9 README.md
- You should see the texts you added being deleted.
- Undo add
- Make sure you are in USERNAME:~/workspace/[name of repo]. If not cd into it.
- Make some changes to your README.md.
- On the terminal, add your README.md to the staging area.
git add README.md
- Your README.md should be showing up as green because it is being added to the staging area.
- Now Check the state of your files
git status
-
Since your README.md is green, you want to unstage it (turn it to red) using the command given above
git reset HEAD README.md
- Now use
git status
, and you should be seeing your README.md unstaged (red).
- Undo commit
- Following from the step above, add README.md to the staging area.
git add README.md
- Your README.md should appear green. Now commit it with a message.
`git commit -m “[text]”
- You have just commited README.md, but you want to undo it. Use the following to help you undo the commit.
git reset --soft HEAD~1
- You have just undo your commit!
- Undo Push
- Make sure you are in USERNAME:~/workspace/[name of repo]. If not cd into it.
- Add some text to README.md
- Add and commit it
- Then
git push
. This will allow you to see your commits in the remote repo.
- At this point, you realized that you did not mean to push it and you want to undo your push. Use the following to undo a push.
- First use
git log
to view the history of your commits.
- Copy the SHA of the commit you want to delete (something like b2322f7df4ada74a0681beb121e6b933fe6b021)
-
Then git revert [SHA]
git revert: undoes a commited snapshop
- Once you git revert, a page will pop up
- Then press control X to exit.